Create Click-to-Run JAR Executable
“When your intuition is strong, follow it.”
— Lolly Daskal
Prologue
When you have a JAR file, you can run it with java -jar JarFile.jar
. However, you may notice that some JAR files are able to run with double-click!😲How is this be done?
In this article, I’m going to talk about how to make your JAR archive that can run with double-click. And perhaps a solution to the most common problem.
Run JAR with Double-click
Creating Executable JAR
To run a JAR, we need to create a JAR first. Duh. Usually, only GUI application may have the need to run with double-click. So here, we write a simplest Swing frame.
1 | import javax.swing.JFrame; |
Now this is it. You can use java Main.java
to test it. Then, let’s make a JAR from it.
First, let’s create a manifest file. Name it anything you like, but manifest.txt
may be a practical choice. It is essential for click-to-run JAR because it tells JVM which class to run.
1 | Main-Class: Main |
Then, use jar
command to create a JAR with manifest. You can add --verbose
or -v
to see the creation process.
1 | jar --create --file=Main.jar --manifest=manifest.txt Main.class |
To make it short, you can use the combo cfm
. Notice that the precedence of f
and m
indicates the order of .jar
target and manifest file. If you switch them by using cmf
, you should also swap the following .jar
and manifest file.
1 | jar cfm Main.jar manifest.txt Main.class |
To add all classes in a folder, you can just use the folder name, or *.class
for top level .class
files.
Now, you’ve created a click-to-run JAR file!🍾
Default Open Action
If it runs as expected, then congratulations!🎊However, things may not go in the right direction.🥲
For the first time, Windows may prompt you to choose the default program to open it. Choose the javaw.exe
of your current environment. Make sure it is of the same version as your javac
. This should work just fine.
javaw
is the same as java
, but will run application without console. So it is usually used to run GUI programs. It is OK to have javaw
from a higher version, but not vice versa.
If it is not the first time, and you’ve configured it some point in the past, things may get a little tricky. Perhaps because of outdated javaw
version, nothing will happen when you double-click a JAR file. Actually, some thing did happen, which is an exception. In this case, you may need to reach out for the registry.
Use Win + R
to open Run dialog, and type regedit
to open Registry Editor. Then, find the following key.
1 | Computer\HKEY_CLASSES_ROOT\jarfile\shell\open\command |
It should look like this. Ensure that the data points to the right javaw
.
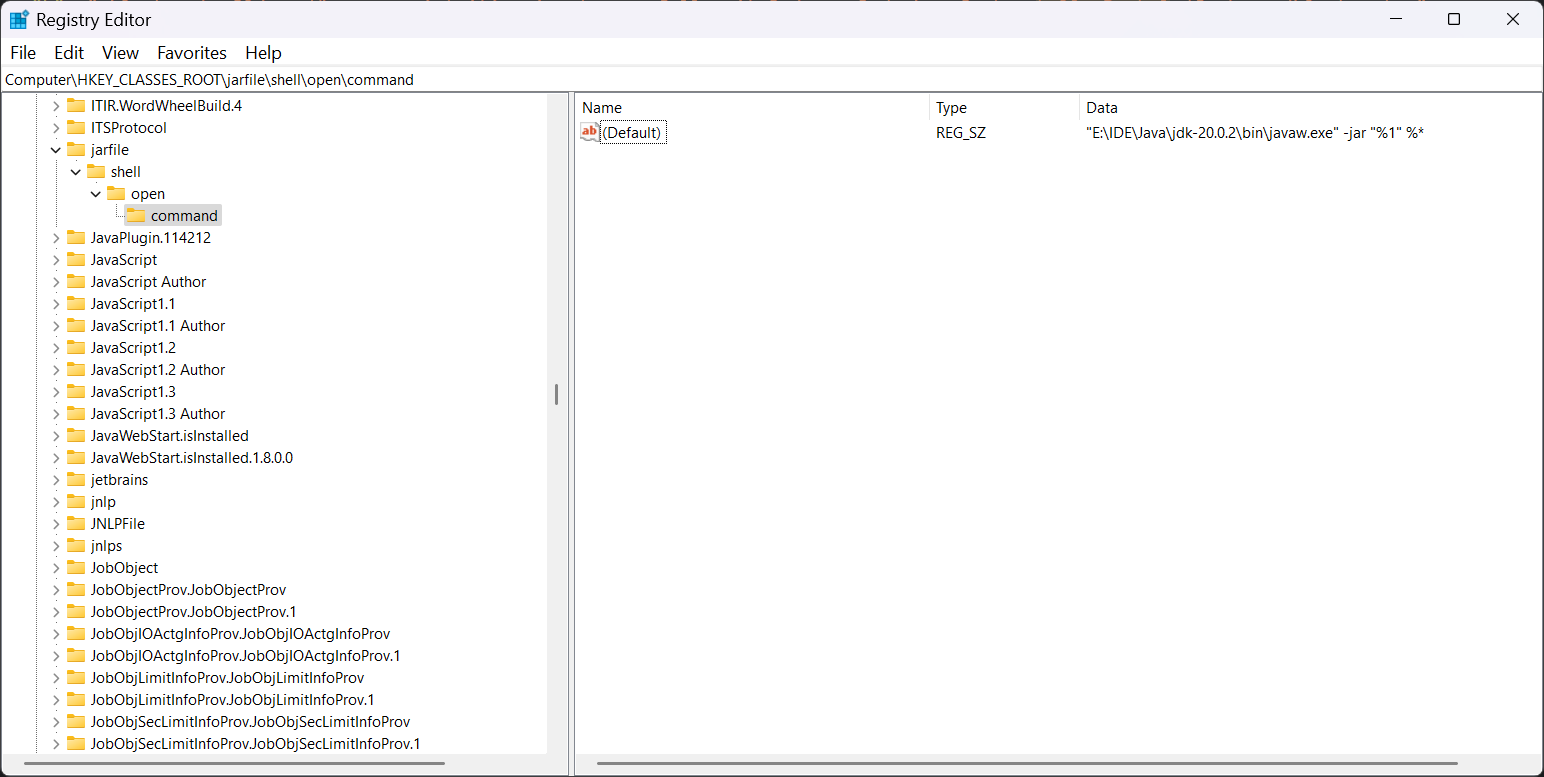
Tada!🎉Now double-click on your JAR file and enjoy!
Epilogue
Well, I guess it is a little trick? It puzzles me for long, and now it’s clear. See you around. ᓚᘏᗢ