Configure Path Aliases in React/Vue With Vite
I learned much from this article:
Why Use Path Aliases
In our React or Vue project, we often import tons of components in our JavaScript files. It would be nice if we use npm
or yarn
to manage then, but… when it comes to our custom ones, the import statements will become something like this: ../../..
. And it would be a nightmare if we want to refactor our file structure.😱
With path aliases, we can use a simple prefix as the root folder of our project, so that import statements could be simpler, and our refactoring will also be much more easier.
Configure Path Aliases
In React/Vue project processed by Vite, things are much easy. And if you create the project with Visual Studio, the front end will be managed by Vite by default.
Although it would be better to write front end code in Visual Studio Code, Visual Studio can create projects based on its templates while managing npm
packages, which is extremely convenient.
First of all, I’d like to give you a brief look of my project structure, which is quite common. Actually you just need to pay attention to src/
folder and vite.config.js
.
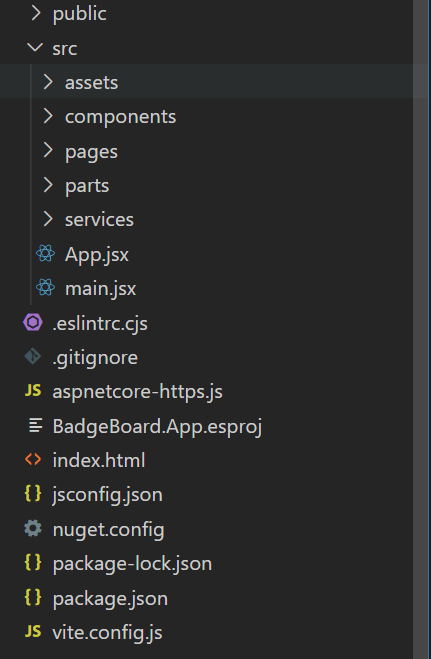
Step 1. Modify Vite Configuration
Well, the primary objective is to make Vite recognize our path aliases. So, open vite.config.js
in our project root, and replace its default resolve to this. The tilde (~
) here is our prefix, and this alias tells Vite to map ~/*
to src/
, while src/
is the a path from vite.config.js
itself.
1 | export default defineConfig({ |
Tilde (~
) is not the only option, in fact, you can use any thing, e.g. @
or whatever you like, as long as it doesn’t mix up with normal pathnames.
Step 2. Use Path Aliases
Now that Vite can recognize our path aliases, we can use it in our import statements. For example, here is my src/
folder structure, and we’re gonna use this ExpandFab
component.
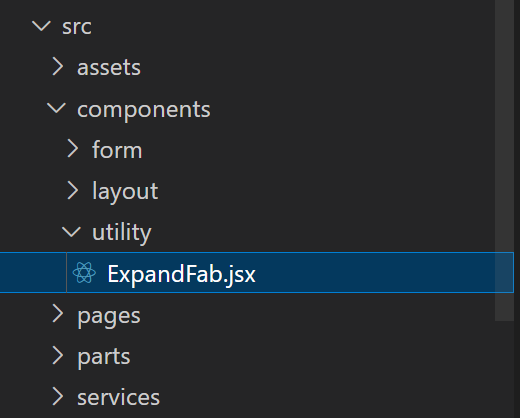
With path aliases, as long as component position not change, we can use the same import statement anywhere. And of course, if you configure alias path more precisely, it is possible to change only the alias mapping when doing refactor.
1 | import ExpandFab from '~/components/utility/ExpandFab'; |
Step 3. Add VSCode Configuration
There is a drawback of path aliases, that code intelliSense may not recognize it.🥴But, if you use Visual Studio Code as your editor, there’s an extra bonus for you.
By simply adding a jsconfig.json
file to your project root, Visual Studio Code can understand what we’re doing. It can be really simple. Just remember to replace the prefix and target path with your own. 😉
1 | { |
Now, Visual Studio Code can recognize your custom aliases.
Epilogue
Well, this is it. Enjoy coding! 🥳
Why! Why Visual Studio could not support these! At least the auto complete for import… The code could have become more robust with much more powerful intelliSense and analyzer. 😣